Python, a versatile and powerful programming language, has become increasingly popular for a wide range of applications, including home automation. With its simple syntax and extensive library support, Python is an excellent choice for creating custom scripts to control smart home devices and automate everyday tasks. In this complete guide, we’ll walk you through the basics of using Python for home automation and provide some examples of Python scripts to get you started.
Why Choose Python for Home Automation?
Python offers several advantages for home automation enthusiasts:
- Easy to learn: Python’s simple syntax and readability make it easy for beginners to pick up and start programming.
- Extensive libraries: Python boasts a vast selection of libraries, making it simple to integrate with various smart home devices and APIs.
- Cross-platform compatibility: Python can run on various platforms, including Windows, macOS, and Linux, ensuring seamless integration with your existing systems.
- Strong community support: Python has a large, active community that offers resources, tutorials, and support for all levels of developers.
Setting Up Your Python Environment
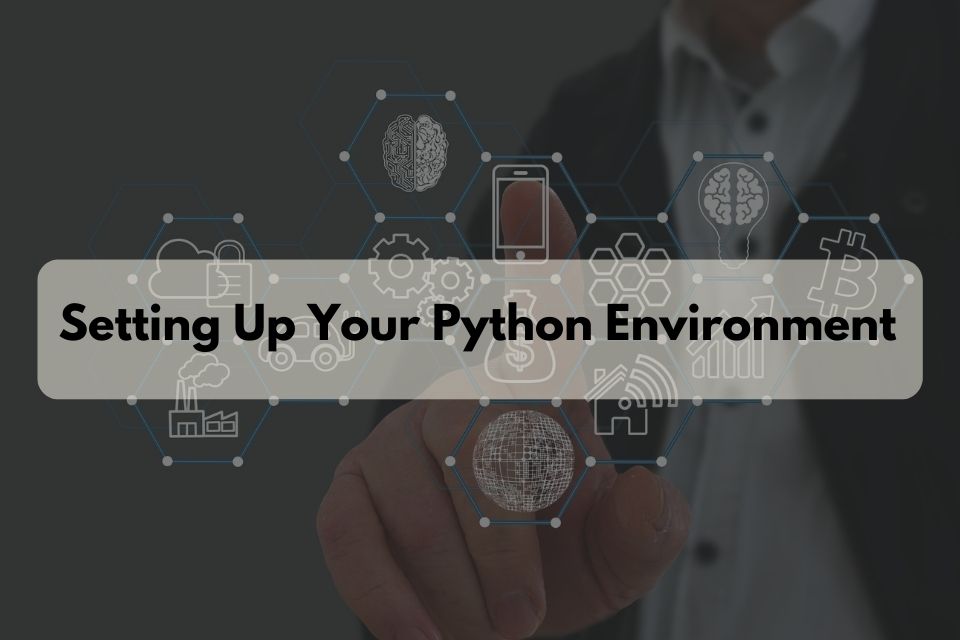
Before diving into Python scripts for home automation, you’ll need to set up your Python environment:
- Download and install the latest version of Python from the official website (https://www.python.org/downloads/). Ensure that you select the appropriate version for your operating system.
- Install an Integrated Development Environment (IDE) for Python, such as Visual Studio Code, PyCharm, or Atom. An IDE will make it easier to write, debug, and manage your Python scripts.
- Familiarize yourself with Python’s syntax and basic programming concepts, such as variables, loops, and functions, through online tutorials or documentation.
Python Libraries for Home Automation
There are numerous Python libraries available to help you interface with various smart home devices and protocols. Some popular libraries include:
- Home Assistant Python API: A Python wrapper for the Home Assistant RESTful API, allowing you to control and automate devices connected to your Home Assistant instance.
- PyHS3: A library for interacting with HomeSeer, a popular home automation software.
- PyZWave: A Python library for controlling Z-Wave devices, a widely used wireless communication protocol for home automation.
- PyInsteon: A Python library for managing Insteon devices, another popular home automation protocol.
- Tuyapy: A Python library for controlling Tuya-based smart devices, such as smart plugs, lights, and switches.
Python Script Examples for Home Automation
Below are some examples of Python scripts you can use to get started with home automation:
a. Control Smart Lights:
import homeassistant_api
api = homeassistant_api.HomeAssistantAPI(“http://your-home-assistant-url:8123”, “your-api-token”)
light_entity_id = “light.living_room”
# Turn on the light
api.call_service(“light”, “turn_on”, {“entity_id”: light_entity_id})
Change the light color
api.call_service(“light”, “turn_on”, {“entity_id”: light_entity_id, “rgb_color”: [255, 0, 0]})
Turn off the light
api.call_service(“light”, “turn_off”, {“entity_id”: light_entity_id})
b. Automate Thermostat:
“`python
import homeassistant_api
api = homeassistant_api.HomeAssistantAPI(“http://your-home-assistant-url:8123”, “your-api-token”)
thermostat_entity_id = “climate.living_room”
# Set the thermostat to a specific temperature
api.call_service(“climate”, “set_temperature”, {“entity_id”: thermostat_entity_id, “temperature”: 72})
c. Monitor Motion Sensors:
import homeassistant_api
api = homeassistant_api.HomeAssistantAPI(“http://your-home-assistant-url:8123”, “your-api-token”)
motion_sensor_entity_id = “binary_sensor.motion_sensor”
def motion_detected(data):
if data[“entity_id”] == motion_sensor_entity_id:
print(“Motion detected!”)
api.listen_state(motion_detected)
Conclusion
Python is a powerful tool for home automation, allowing you to create custom scripts that can control and automate your smart home devices. By leveraging Python’s simplicity, extensive library support, and cross-platform compatibility, you can enhance your home automation experience and create a truly intelligent living space. Start exploring Python for home automation today and unlock the full potential of your smart home devices.
Frequently Asked Questions and Answers
- What is home automation?
Home automation refers to the use of technology to control and automate everyday tasks in your home, such as adjusting lighting, controlling appliances, managing temperature, and monitoring security systems.
- Can Python be used for home automation?
Yes, Python is an excellent programming language for home automation due to its simplicity, extensive library support, and cross-platform compatibility. It allows you to create custom scripts to control and automate various smart home devices.
- How do I start using Python for home automation?
To start using Python for home automation, you need to set up your Python environment by downloading and installing the latest version of Python, choosing an Integrated Development Environment (IDE), and familiarizing yourself with Python’s syntax and basic programming concepts.
- Which Python libraries can I use for home automation?
There are numerous Python libraries available for home automation, including Home Assistant Python API, PyHS3, PyZWave, PyInsteon, and Tuyapy, among others. These libraries help you interface with various smart home devices and protocols.
- Can Python control smart lights?
Yes, Python can control smart lights using appropriate libraries, such as Home Assistant Python API or Tuyapy, depending on your smart light’s compatibility.
- Can I use Python to automate my thermostat?
Yes, you can use Python to automate your thermostat by utilizing relevant libraries, like the Home Assistant Python API, and creating custom scripts to adjust the temperature or switch between heating and cooling modes.
- How do I monitor motion sensors with Python?
To monitor motion sensors with Python, you can use libraries like Home Assistant Python API to listen for changes in the motion sensor’s state and trigger custom actions or notifications when motion is detected.
- Can Python be used to control smart plugs and switches?
Yes, Python can be used to control smart plugs and switches using appropriate libraries, such as Tuyapy for Tuya-based devices, or Home Assistant Python API for devices connected to your Home Assistant instance.
- Is Python suitable for beginners in home automation?
Python is an excellent choice for beginners in home automation due to its easy-to-learn syntax, extensive library support, and strong community resources, which provide a wealth of tutorials and examples to help you get started.
- Can I use Python for home automation on different operating systems?
Yes, Python is a cross-platform programming language that can run on various operating systems, including Windows, macOS, and Linux, ensuring seamless integration with your existing systems.
- Can I integrate Python with voice assistants like Amazon Alexa or Google Assistant?
Yes, you can integrate Python with voice assistants like Amazon Alexa or Google Assistant using libraries and APIs provided by these platforms, allowing you to control your smart home devices with voice commands.
- How do I create a custom Python script for home automation?
To create a custom Python script for home automation, you’ll need to choose a suitable library for your devices, import the library into your Python script, and write functions to control and automate your smart home devices.
- Can Python control smart home security systems?
Yes, Python can be used to control and monitor smart home security systems, such as cameras, door/window sensors, and alarm systems, using appropriate libraries and APIs.
- How do I connect my Python script to my smart home devices?
To connect your Python script to your smart home devices, you will need to use appropriate libraries and APIs compatible with your devices. These libraries often require device-specific configurations, such as API tokens, IP addresses, or authentication credentials, to establish a connection.
- Can I automate multiple devices with a single Python script?
Yes, you can automate multiple devices with a single Python script by importing the necessary libraries for each device type and writing functions to control and automate each device.
- Can I use Python to create custom home automation routines?
Yes, you can use Python to create custom home automation routines by writing scripts that combine multiple device controls, perform conditional actions, and execute tasks based on time or events.
- Can I use Python to create a web interface for my home automation system?
Yes, you can use Python to create a web interface for your home automation system by leveraging web frameworks like Flask or Django, which allow you to build web applications that interact with your Python scripts and smart home devices.
- Are there any security concerns when using Python for home automation?
As with any technology, there can be security concerns when using Python for home automation. To minimize risks, ensure you use secure authentication methods, keep your Python environment and libraries up-to-date, and follow best practices for securing your smart home devices and network.
- Can I schedule Python scripts to run at specific times for home automation?
Yes, you can schedule Python scripts to run at specific times using tools like cron (for Linux and macOS) or Task Scheduler (for Windows), enabling time-based home automation routines.
- Can I use Python to monitor energy consumption in my smart home?
Yes, you can use Python to monitor energy consumption in your smart home by connecting to compatible smart energy meters or devices that report power usage data. Utilize appropriate libraries and APIs to collect this data and analyze it within your Python script. You can then use this information to optimize your home’s energy consumption and identify potential areas for improvement.